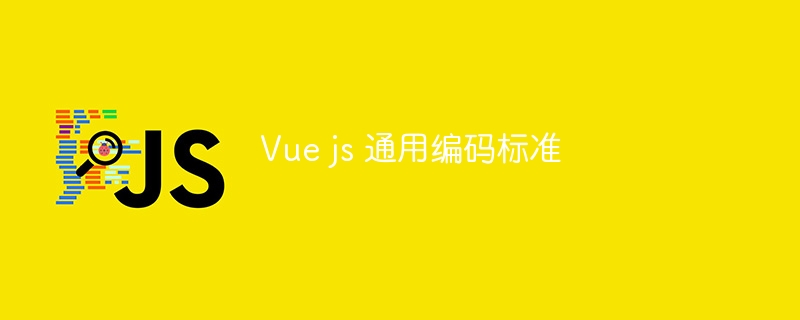
以下是 vue.JS 的其他好的和坏的做法:
- 避免魔法数字和字符串:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
const max_items = 10;
function additem(item) {
if (items.length
<ol>
<li>
<strong>高效使用 v- for :</strong>
<ul>
<li>使用 v- for 时,始终提供唯一的键来优化渲染。
</li>
</ul>
</li>
</ol><pre class = "brush:php;toolbar:false" > <!-- good -->
<div v- for = "item in items" :key= "item.id" >
{{ item.name }}
</div>
<!-- bad -->
<div v- for = "item in items" >
{{ item.name }}
</div>
|
- 避免内联样式:
- 更喜欢使用 CSS 类而不是内联样式,以获得更好的可维护性。
1
2
3
4
5
6
7
8
|
<!-- good -->
<div class = "item" >{{ item.name }}</div>
<style scoped>
.item {
color: red;
}
</style><!-- bad --><div :style= "{ color: 'red' }" >{{ item.name }}</div>
|
组件实践
- 组件可重用性:
1
2
3
4
5
|
<basebutton :label= "buttonlabel" :disabled= "isdisabled" ></basebutton>
<button :disabled= "isdisabled" >{{ buttonlabel }}</button>
|
- 道具验证:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
props: {
title: {
type: string,
required: true
},
age: {
type: number,
default : 0
}
}
props: {
title: string,
age: number
}
|
- 避免长方法:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
methods: {
fetchdata() {
this.fetchuserdata();
this.fetchpostsdata();
},
async fetchuserdata() { ... },
async fetchpostsdata() { ... }
}
methods: {
async fetchdata() {
const userresponse = await fetch( 'API/user' );
this.user = await userresponse.JSON();
const postsresponse = await fetch( 'api/posts' );
this.posts = await postsresponse.json();
}
}
|
- 避免具有副作用的计算属性:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
computed: {
fullname() {
return `${this.firstname} ${this.lastname}`;
}
}
computed: {
fetchdata() {
this.fetchuserdata();
return this.user;
}
}
|
模板实践
- 使用 v-show 与 v-if:
- 使用 v-show 来切换可见性,而无需从 dom 添加/删除元素,并在有条件渲染元素时使用 v-if。
1
2
3
4
5
6
7
8
|
<!-- good: use v-show for toggling visibility -->
<div v-show= "isvisible" >content</div>
<!-- good: use v- if for conditional rendering -->
<div v- if = "isloaded" >content</div>
<!-- bad: use v- if for simple visibility toggling -->
<div v- if = "isvisible" >content</div>
|
- 避免使用大模板:
- 保持模板干净、小;如果它们变得太大,请将它们分解成更小的组件。
1
2
3
4
5
6
7
8
|
<!-- good: small, focused template -->
<template><div>
<baseHEADer></baseheader><basecontent></basecontent><basefooter></basefooter>
</div>
</template><!-- bad: large, monolithic template --><template><div>
<header>...</header><main>...</main><footer>...</footer>
</div>
</template>
|
状态管理实践
- 使用vuex进行状态管理:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
export default new vuex.store({
state: { user: {} },
mutations: {
setuser(state, user) {
state.user = user;
}
},
actions: {
async fetchuser({ commit }) {
const user = await fetchuserdata();
commit( 'setuser' , user);
}
}
});
|
- 避免组件中的直接状态突变:
- 使用突变来修改 vuex 状态,而不是直接突变组件中的状态。
1
2
3
4
5
6
7
8
9
10
11
12
13
|
methods: {
updateuser() {
this. $store .commit( 'setuser' , newuser);
}
}
methods: {
updateuser() {
this. $store .state.user = newuser;
}
}
|
错误处理和调试
- 全局错误处理:
- 使用 vue 的全局错误处理程序来捕获和处理错误。
1
2
3
|
vue.config.errorhandler = function (err, vm, info) {
console.error( 'vue error:' , err);
};
|
- 提供用户反馈:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
methods: {
async fetchData() {
try {
const data = await fetchData();
this.data = data;
} catch (error) {
this.errORMessage = 'Failed to load data. Please try again.' ;
}
}
}
methods: {
async fetchData() {
try {
this.data = await fetchData();
} catch (error) {
console.error( 'Error fetching data:' , error);
}
}
}
|
通过遵循这些额外的实践,您可以进一步提高 vue.js 应用程序的质量、可维护性和效率。