go 中集成 orientdb 数据库:安装 orientdb 客户端库和 orientdb。初始化客户端,指定服务器地址、身份验证信息和目标数据库。使用 sql 查询数据库并处理查询结果。创建新文档并检索其 id。通过 id 读入现有文档,更新其字段,并保存更改。通过 id 删除文档。
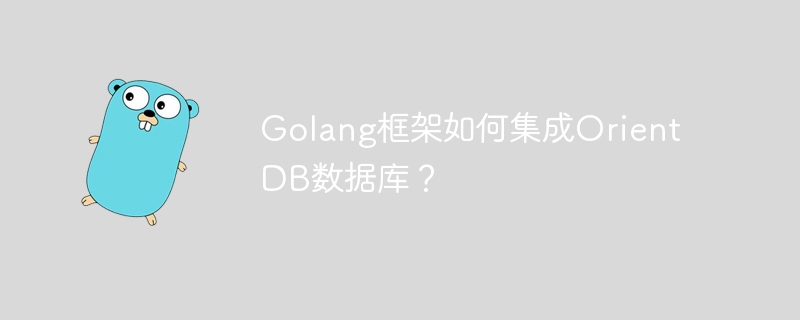
如何在 Go 中集成 OrientDB 数据库
OrientDB 是一款 NoSQL 多模型数据库,以其高性能、灵活的数据模型和易用性而闻名。本教程将介绍如何在 Go 应用程序中集成 OrientDB。
先决条件
- 安装 Golang
- 安装 OrientDB
- OrientDB 仪表板用户名和密码
步骤
1. 安装 OrientDB 客户端库
1
|
go get Github.com/orientechnologies/orientdb- go
|
2. 初始化客户端
立即学习“go语言免费学习笔记(深入)”;
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
import (
"context"
"fmt"
orientdb "github.com/orientechnologies/orientdb-go"
)
func main() {
client, err := orientdb.NewClient(
orientdb.NewAddress( "localhost" , 2424 ),
orientdb.NewAuthBasic( "root" , "root" ),
orientdb.Newdatabase( "test" ),
)
if err != nil {
panic (err)
}
defer client. Close ()
}
|
3. 查询数据库
1
2
3
4
5
6
7
8
9
|
query := ` SELECT * FROM Person`
results, err := client.Read(context.Background(), query)
if err != nil {
panic (err)
}
for _, record := range results {
fmt. Println (record.GetField( "name" ))
}
|
4. 创建文档
1
2
3
4
5
6
7
8
9
10
|
newDocument := map [ string ] interface {}{
"name" : "John Doe" ,
"age" : 30 ,
}
record, err := client.Create(context.Background(), "Person" , newDocument)
if err != nil {
panic (err)
}
fmt. Println (record.GetField( "name" ))
|
5. 更新文档
1
2
3
4
5
6
7
8
9
10
|
record, err := client.Read(context.Background(), "Person" , record.ID)
if err != nil {
panic (err)
}
record.SetField( "name" , "Jane Doe" )
err = client.Update(context.Background(), record)
if err != nil {
panic (err)
}
|
6. 删除文档
1
2
3
4
|
err = client. Delete (context.Background(), "Person" , record.ID)
if err != nil {
panic (err)
}
|