使用go语言框架集成elasticsearch数据库涉及以下步骤:安装elasticsearch客户端库、创建客户端、创建索引、索引文档、搜索文档、更新文档和删除文档。客户端配置包括地址、用户名和密码。创建索引需要指定索引名称和映射。索引文档需要提供索引名称、文档id和文档数据。搜索文档需要指定查询条件。更新文档需要提供文档id和更新数据。删除文档需要提供索引名称和文档id。
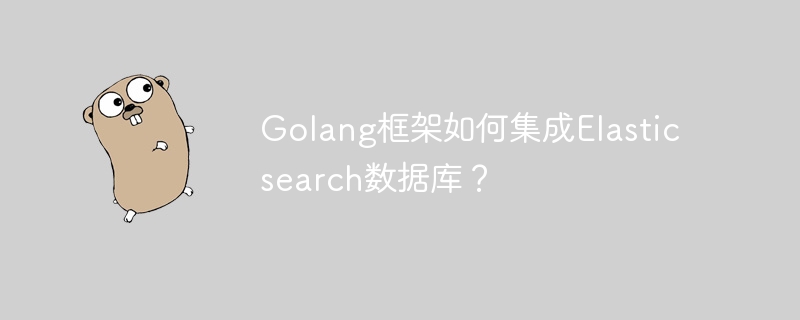
利用Go语言框架集成Elasticsearch数据库
简介
Elasticsearch是一个流行的开源分布式搜索引擎,为海量数据集提供了强大的搜索和分析功能。本文将介绍如何使用Go语言框架将Elasticsearch集成到你的应用程序中。
立即学习“go语言免费学习笔记(深入)”;
先决条件
- Go 1.16或更高版本
- Elasticsearch 7.0或更高版本
安装依赖项
首先,安装Go语言Elasticsearch客户端库:
1
|
go get Github.com/elastic/ go -elasticsearch/v8
|
连接到Elasticsearch
使用elasticsearch.NewClient函数创建一个新的客户端,该函数接受一个配置对象作为参数:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
import (
"context"
"fmt"
"time"
"github.com/elastic/go-elasticsearch/v8"
)
func main() {
cfg := elasticsearch.Config{
Addresses: [] string { "localhost:9200" },
username: "elastic" ,
Password: "changeme" ,
}
client, err := elasticsearch.NewClient(cfg)
if err != nil {
panic (err)
}
ctx := context.Background()
res, err := client.Ping(ctx).Do()
if err != nil {
panic (err)
}
fmt. Println (res)
}
|
创建一个索引
将数据存储在Elasticsearch中需要索引。可以使用Create函数创建一个索引:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
func createIndex(client *elasticsearch.Client) {
ctx := context.Background()
req := elasticsearch.CreateIndexRequest{
Index: "my_index" ,
Body: [] byte (`
{
"mappings" : {
"properties" : {
"name" : {
"type" : "text"
},
"age" : {
"type" : "integer"
}
}
}
}
`),
}
res, err := client.CreateIndex(req).Do(ctx)
if err != nil {
panic (err)
}
fmt. Println (res)
}
|
索引文档
将数据添加到Elasticsearch需要索引文档。可以使用Index函数添加文档:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
func indexDocument(client *elasticsearch.Client) {
ctx := context.Background()
req := elasticsearch.IndexRequest{
Index: "my_index" ,
DocumentID: elasticsearch.RandString( 32 ),
BodyJSON: map [ string ] interface {}{
"name" : "John Doe" ,
"age" : 30 ,
},
}
res, err := client.Index(req).Do(ctx)
if err != nil {
panic (err)
}
fmt. Println (res)
}
|
搜索文档
可以使用Search函数搜索Elasticsearch中的文档:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
func searchDocuments(client *elasticsearch.Client) {
ctx := context.Background()
req := elasticsearch.SearchRequest{
Index: "my_index" ,
BodyJSON: map [ string ] interface {}{
"query" : map [ string ] interface {}{
"match" : map [ string ] interface {}{
"name" : "John Doe" ,
},
},
},
}
res, err := client.Search(req).Do(ctx)
if err != nil {
panic (err)
}
for _, hit := range res.Hits.Hits {
fmt. Println (hit.Source)
}
}
|
更新文档
可以使用Update函数更新Elasticsearch中的文档:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
func updateDocument(client *elasticsearch.Client) {
ctx := context.Background()
req := elasticsearch.UpdateRequest{
Index: "my_index" ,
DocumentID: "1" ,
BodyJSON: map [ string ] interface {}{
"doc" : map [ string ] interface {}{
"age" : 31 ,
},
},
}
res, err := client.Update(req).Do(ctx)
if err != nil {
panic (err)
}
fmt. Println (res)
}
|
删除文档
可以使用Delete函数删除Elasticsearch中的文档:
1
2
3
4
5
6
7
8
9
10
11
12
13
|
func deleteDocument(client *elasticsearch.Client) {
ctx := context.Background()
req := elasticsearch.DeleteRequest{
Index: "my_index" ,
DocumentID: "1" ,
}
res, err := client. Delete (req).Do(ctx)
if err != nil {
panic (err)
}
fmt. Println (res)
}
|