go框架通过提供多种工具优化应用程序性能,包括:缓存,将频繁访问的数据存储在快速访问位置,减少对数据库的调用。数据库管理,优化数据库连接池,提升查询效率。并发和并行,利用goroutine和通道同时处理多个请求。监控和指标,收集和报告指标数据,以便识别性能瓶颈。
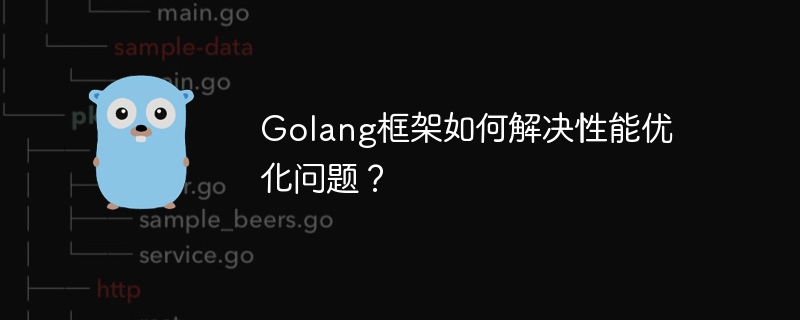
Go框架中的性能优化
高性能是现代Web应用程序的关键方面。Go语言以其出色的性能而闻名,而其框架更进一步,提供了广泛的工具来优化应用程序的效率。
缓存
立即学习“go语言免费学习笔记(深入)”;
缓存是一种将频繁访问的数据存储在快速访问位置的机制。Go框架,例如Gin,提供中间件,您可以使用中间件将处理程序函数的结果存储在缓存中。这减少了对数据库或其他数据源的调用,提高了性能。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
|
import (
"fmt"
"net/HTTP"
"Github.com/gin-gonic/gin"
)
func main() {
r := gin. Default ()
data := map [ string ] string {
"key1" : "value1" ,
"key2" : "value2" ,
}
r.GET( "/" , gin.Cache( 10 ), func (c *gin.Context) {
key := c.Query( "key" )
if value, ok := data[key]; ok {
c.JSON(http.StatusOK, gin.H{
"key" : key,
"value" : value,
})
} else {
c.JSON(http.StatusNotFound, gin.H{
"error" : "Key not found" ,
})
}
})
r.Run()
}
|
数据库管理
Go框架提供了对底层数据库连接池的访问。这可以优化数据库交互,减少打开和关闭数据库连接的开销。例如,GORM库提供了连接池支持,从而提高了查询效率。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
import (
"fmt"
"time"
"github.com/jinzhu/gORM"
_ "github.com/jinzhu/gorm/dialects/postgres"
)
func main() {
db, err := gorm.Open( "postgres" , "user=postgres password=mypassword dbname=mydb SSLmode=disable" )
if err != nil {
panic (err)
}
db.DB().SetMaxIdleConns( 10 )
db.DB().SetMaxOpenConns( 10 )
db.DB().SetConnMaxLifetime(time.Hour)
var users []User
if err := db.Find(&users). Error ; err != nil {
panic (err)
}
for _, user := range users {
fmt. Println (user.Name)
}
}
|
并发和并行
Go的并发特性使用户可以编写同时处理多个请求的应用程序。这可以通过使用Goroutine和通道实现。例如,Echo框架提供了异步处理程序,允许同时处理多个请求。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
import (
"fmt"
"net/http"
"github.com/labstack/echo/v4"
)
func main() {
e := echo. New ()
e.GET( "/async" , func (c echo.Context) error {
go func () {
fmt. Println ( "异步任务开始" )
fmt. Println ( "异步任务结束" )
}()
return c. String (http.StatusOK, "OK" )
})
e.Start( ":8080" )
}
|
监控和指标
监控和指标对于了解应用程序的性能至关重要。Go框架,例如Prometheus,提供工具来收集和报告指标,例如内存使用、请求持续时间和错误率。这使开发人员能够识别性能瓶颈并采取措施进行优化。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
|
import (
"fmt"
"github.com/prometheus/client_golang/prometheus"
"github.com/prometheus/client_golang/prometheus/promhttp"
"net/http"
)
var requestLatency = prometheus.NewHistogramVec(prometheus.HistogramOpts{
Name: "http_request_latency_seconds" ,
Help: "Histogram of request latencies." ,
Buckets: prometheus.ExponentialBuckets( 0.001 , 2 , 10 ),
}, [] string { "route" })
func main() {
prometheus.MustRegister(requestLatency)
http.HandleFunc( "/" , func (w http.ResponseWriter, r *http.Request) {
latency := prometheus.NewTimer(requestLatency.WithLabelValues(r.URL.Path))
fmt.Fprintf(w, "Hello, World!" )
latency.ObserveDuration()
})
http.Handle( "/metrics" , promhttp.Handler())
http.ListeNANDServe( ":8080" , nil )
}
|
通过使用Go框架中可用的工具,开发人员可以有效地优化应用程序的性能,以满足现代Web应用程序的高要求。