c++++ 中,异常用于处理运行时错误。通过 try-catch-throw 机制,函数可抛出异常以报告错误,而调用者可通过 catch 块处理特定异常或所有异常。例如,一个读取文件并计算行数的函数可能会抛出一个异常,指示文件不存在或无法打开。
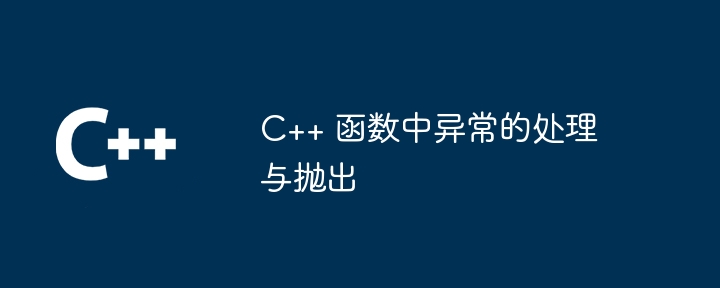
C++ 函数中异常的处理与抛出
在 C++ 中,异常是处理运行时错误的强大机制。当函数在执行期间遇到意外情况时,可以使用异常来表示错误。
异常处理
立即学习“C++免费学习笔记(深入)”;
要处理函数中的异常,可以使用 try、catch 和 throw 关键字。
- try:将可能引发异常的代码放在 try 块中。
- catch:处理特定类型异常的代码放在 catch 块中。
- throw:在函数中抛出一个异常。
例如:
1
2
3
4
5
6
7
8
9
|
void someFunction() {
try {
} catch ( const std::exception& e) {
} catch (...) {
}
}
|
异常抛出
使用 throw 关键字抛出异常。该关键字后跟一个表示错误的异常对象。
例如:
1
2
3
|
void someFunction() {
throw std::runtime_error( "Some error occurred" );
}
|
实战案例
考虑一个读取文件并计算其行数的函数。如果文件不存在或无法打开,函数应抛出一个异常。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
int countLines( const std::string& filename) {
std::ifstream file(filename);
if (!file.is_open()) {
throw std::invalid_argument( "File could not be opened" );
}
int lineCount = 0;
std::string line;
while (std::getline(file, line)) {
lineCount++;
}
return lineCount;
}
|
在 main 函数中,我们可以在 try 块中调用此函数,并在 catch 块中处理异常:
1
2
3
4
5
6
7
8
|
int main() {
try {
int lineCount = countLines( "some_file.txt" );
std::cout << "Line count: " << lineCount << std::endl;
} catch ( const std::invalid_argument& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
}
|