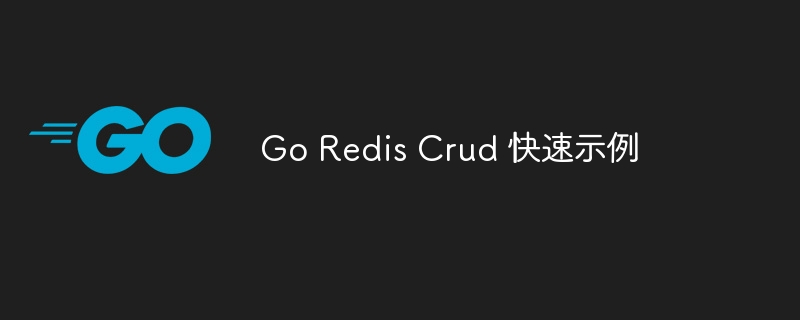
安装依赖和环境变量
将数据库连接中的值替换为您的值。
1
2
3
4
5
6
7
8
|
.env file
Redis_address=localhost
redis_port=6379
redis_password=123456
redis_db=0
#install on go
go get Github.com/redis/go-redis/v9
|
redis管理器
创建一个文件来管理.go 这将包含一个方法来获取与redis的连接,例如在其他模块和服务中。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
|
package main
import (
"fmt"
"github.com/redis/go-redis/v9"
"os"
"strconv"
)
const customerdb = 0
type redismanager struct {
db int
client *redis.client
}
func newredisclient(customerdb int) (*redismanager, error) {
address := os. getenv ( "redis_address" )
if address == "" {
return nil, fmt.errorf( "redis_address is not set" )
}
password := os. getenv ( "redis_password" )
if password == "" {
return nil, fmt.errorf( "redis_password is not set" )
}
port := os. getenv ( "redis_port" )
if port == " " {
return nil, fmt.errorf( "redis_port is not set" )
}
db := os. getenv ( "redis_db" )
if db == "" {
return nil, fmt.errorf( "redis_db is not set" )
}
redisdb, err := strconv.atoi(db)
if err != nil {
return nil, fmt.errorf( "redis_db is not a number" )
}
cli := redis.newclient(&redis.options{
addr: fmt.sprintf( "%s:%s" , address, port),
password: password,
db: redisdb,
})
return &redismanager{
client: cli,
db: customerdb,
}, nil
}
func (rd *redismanager) setdb(db int) {
rd.db = db
}
|
创建用于管理实体(客户)存储库的结构
创建一个结构体来管理redis连接并获取与redis实体交互的所有方法(crud操作和查询)
有了这个结构,任何时候我们需要访问实体(客户)数据,我们都可以实例化并开始将其用作存储库模式。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
type customerrepo struct {
cli *redismanager
db int
}
func newcustomerrepo() (*customerrepo, error) {
cli, err := newredisclient(customerdb)
if err != nil {
return nil, err
}
return &customerrepo{
cli: cli,
}, nil
}
|
创建结构体实体
在客户实体上添加与面包字段映射的标签。
redis:“-”与要保存在redis上的字段解除关系。如果您想要一个文件或结构不保存,请不要添加标签。
1
2
3
4
5
6
7
|
type customer struct {
id string `redis: "id" `
name string `redis: "name" `
email string `redis: "email" `
phone string `redis: "phone" `
age int `redis: "age" `
}
|
增删改查方法
存储、更新或从实体获取信息的方法示例。
这些方法是从 customersrepo 实体使用的。
他们收到包含信息的客户实体,并根据操作返回结果。
1
2
3
|
func (c *customerrepo) save(customer *customer) error {
return c.cli.client.hset(context.todo(), customer.id, customer).err()
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
func (c *customerrepo) get(id string) (*customer, error) {
customer := &customer{}
resmap := c.cli.client.hgetall(context.todo(), id)
if resmap.err() != nil {
return nil, resmap.err()
}
if len(resmap.val()) == 0 {
return nil, nil
}
err := resmap.scan(customer)
if err != nil {
return nil, err
}
return customer, nil
}
|
更新一条新记录
1
2
3
|
func (c *customerrepo) update(customer *customer) error {
return c.cli.client.hset(context.todo(), customer.id, customer).err()
}
|
删除一条新记录
1
2
3
|
func (c *CustomerRepo) Delete (id string) error {
return c.Cli.Client.Del(context.TODO(), id).Err()
}
|
redis 测试示例