如何提升 golang 项目性能和可靠性?使用 goroutine 和 channels 等并发模式提升性能。优化 grpc 服务,如启用 grpc 压缩。启用内存分配跟踪来识别内存泄漏。选择适当的日志记录级别以减少日志噪声。使用基准测试和性能分析来识别性能瓶颈并评估优化结果。
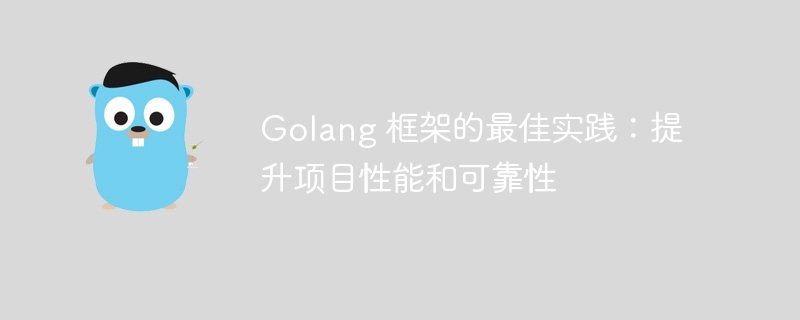
Golang 框架最佳实践:提升项目性能和可靠性
1. 使用适当的并发模式
Golang 提供强大的并发特性。选择合适的并发模式,例如 goroutine 和 channels,可以提升程序性能。
立即学习“go语言免费学习笔记(深入)”;
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
|
package main
import (
"context"
"fmt"
"sync"
)
type safeGoroutinePool struct {
pool *sync.Pool
}
func main() {
pool := &safeGoroutinePool{&sync.Pool{ New : func () interface {} { return &worker{}}}}
ctx, cancel := context.WithCancel(context.Background())
workers := 10
for i := 0 ; i < workers; i++ {
pool.pool.Put(pool.pool. New ())
}
for i := 0 ; i < workers; i++ {
x := i
go func () {
defer pool.pool.Put(pool.pool.Get())
for {
select {
case <-ctx.Done():
return
default :
work(x)
}
}
}()
}
cancel()
}
|
2. 优化 gRPC 服务
gRPC 是构建分布式服务的流行框架。通过以下优化提升 gRPC 服务的性能:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
|
package main
import (
"context"
"google.golang.org/grpc"
"google.golang.org/grpc/codes"
"google.golang.org/grpc/metadata"
"google.golang.org/protobuf/proto"
)
func grpcRequestCompression() grpc.UnaryServerInterceptor {
return func (ctx context.Context, req interface {}, info *grpc.UnaryServerInfo, handler grpc.UnaryHandler) ( interface {}, error ) {
md, ok := metadata.FromIncomingContext(ctx)
if !ok {
return nil , grpc.Errorf(codes.InvalidArgument, "Missing metadata" )
}
ce := md.Get( "grpc-encoding" )
if len (ce) > 0 && ce[ 0 ] == "gzip" {
resp, err := handler(ctx, req)
if err != nil {
return nil , err
}
if msg, ok := resp.(proto.Message); ok {
b, err := proto.Marshal(msg)
if err != nil {
return nil , err
}
return &response{Data: b, ContentEncoding: "gzip" }, nil
}
}
return handler(ctx, req)
}
}
|
3. 启用内存分配跟踪
通过启用内存分配跟踪,可以识别程序中的内存泄漏和其他内存问题。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
|
package main
import (
"fmt"
"log"
"net/http"
"runtime"
"runtime/pprof"
"time"
)
func main() {
runtime.SetBlockProfileRate( 2 )
go func () {
for {
slice := make ([] byte , 1024 )
runtime.SetFinalizer(&slice, func (*[] byte ) { fmt. Println ( "Finalizer called" ) })
}
}()
http.HandleFunc( "/" , func (w http.ResponseWriter, r *http.Request) {
w.Header().Set( "Content-Type" , "text/plain" )
p := pprof.Lookup( "block" )
p.WriteTo(w, 0 )
})
log.Fatal(http.ListenAndServe( "localhost:8080" , nil ))
}
|
4. 使用适当的日志记录级别
选择适当的日志记录级别,可以减少日志噪声并提高调试效率。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
package main
import (
"log"
)
func main() {
log.SetFlags(log.Ldate | log.Ltime | log.Lmicroseconds | log.Llongfile)
log. Println ( "This is a trace-level log" )
log.SetFlags( 0 )
log. Println ( "This is a debug-level log" )
log.Printf( "This is a info-level log: %s" , "some info" )
log.Printf( "This is a warn-level log: %s" , "some warning" )
log.Printf( "This is a error-level log: %s" , "some error" )
}
|
5. 进行基准测试和性能分析
基准测试和性能分析可以帮助识别性能瓶颈并评估优化措施。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
|
package main
import (
"context"
"fmt"
"net/http"
"testing"
"time"
"runtime"
)
func main() {
http.HandleFunc( "/" , func (w http.ResponseWriter, r *http.Request) {
ctx := context.Background()
startTime := time.Now()
fmt.Fprintf(w, "请求处理完成, 用时: %s" , time.Since(startTime))
})
log.Fatal(http.ListenAndServe( "localhost:8080" , nil ))
}
func BenchmarkHandler(b *testing.B) {
for n := 0 ; n < b.N; n++ {
r := http.Request{}
var w http.ResponseWriter
handler(&w, &r)
}
}
func TestPerformance(t *testing.T) {
result := testing.Benchmark( func (b *testing.B) {
for n := 0 ; n < b.N; n++ {
r := http.Request{}
var w http.ResponseWriter
handler(&w, &r)
}
})
fmt.Printf( "处理一个请求平均耗时: %s\n" , result.NsPerOp()/ 1000000 )
fmt.Printf( "运行过程中go程最大数量: %d\n" , runtime.NumGoroutine())
}
|
登录后复制