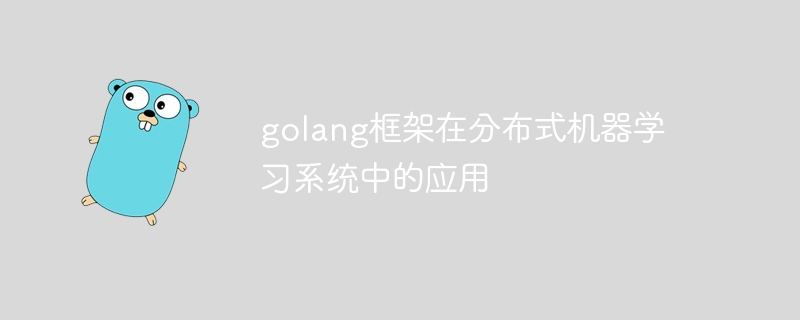
Golang 框架在分布式机器学习系统中的应用
引言
分布式机器学习系统是处理大规模数据集的强有力工具。Golang 以其并发性、易用性和丰富的库而闻名,使其成为构建此类系统的理想选择。本文探讨了 Golang 框架在分布式机器学习系统中的应用,并提供了实战案例。
Go 框架
立即学习“go语言免费学习笔记(深入)”;
- gRPC:一个高性能 RPC 框架,适合分布式系统间通信。
- Celery:一个分布式任务队列,用于处理异步任务。
- Kubernetes:一个容器编排系统,用于管理和调度容器化应用程序。
实战案例
使用 gRPC 构建分布式训练系统
使用 gRPC 创建一个包含工作者和参数服务器的分布式训练系统。工作者负责训练模型,而参数服务器负责聚合梯度。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
|
package main
import (
"context"
"github.com/grpc/grpc-go"
pb "github.com/example/ml/proto"
)
func main() {
conn, err := grpc.Dial( "localhost:50051" , grpc.WithInsecure())
if err != nil {
panic (err)
}
defer conn. Close ()
client := pb.NewParameterServerClient(conn)
params := &pb.Parameters{
W: [] float32 { 0.1 , 0.2 },
B: [] float32 { 0.3 },
}
gradients, err := client.Train(context.Background(), &pb.TrainingRequest{
Params: params,
})
if err != nil {
panic (err)
}
params.W[ 0 ] += gradients.W[ 0 ]
params.W[ 1 ] += gradients.W[ 1 ]
params.B[ 0 ] += gradients.B[ 0 ]
}
package main
import (
"context"
"github.com/grpc/grpc-go"
pb "github.com/example/ml/proto"
)
func main() {
lis, err := net.Listen( "tcp" , "localhost:50051" )
if err != nil {
panic (err)
}
s := grpc.NewServer()
pb.RegisterParameterServer(s, &Server{})
if err := s.Serve(lis); err != nil {
panic (err)
}
}
type Server struct {
mu sync.Mutex
}
func (s *Server) Train(ctx context.Context, req *pb.TrainingRequest) (*pb.TrainingResponse, error ) {
s.mu.Lock()
defer s.mu.Unlock()
res := &pb.TrainingResponse{
Gradients: &pb.Gradients{
W: [] float32 {- 1 , - 1 },
B: [] float32 {- 1 },
},
}
return res, nil
}
|
使用 Celery 构建异步数据处理管道
使用 Celery 创建一个异步数据处理管道,将原始数据转换为训练数据。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
from celery import Celery
celery = Celery(
"tasks" ,
broker= "redis://localhost:6379" ,
backend= "redis://localhost:6379"
)
@celery.task
def preprocess_data(raw_data):
# 预处理原始数据
# ...
return processed_data
|
使用 Kubernetes 部署分布式机器学习系统
使用 Kubernetes 部署分布式机器学习系统,其中工作者和参数服务器作为容器运行。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
|
apiVersion: apps/v1
kind: Deployment
metadata:
name: worker-deployment
spec:
selector:
matchLabels:
app: worker
template:
metadata:
labels:
app: worker
spec:
containers:
- name: worker
image: my-worker-image
command: [ "./worker" ]
args: [ "--param-server-addr=my-param-server" ]
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: parameter-server-deployment
spec:
selector:
matchLabels:
app: parameter-server
template:
metadata:
labels:
app: parameter-server
spec:
containers:
- name: parameter-server
image: my-parameter-server-image
command: [ "./parameter-server" ]
|
登录后复制