在高并发场景中,golang 框架的性能优化策略包括:并行处理:使用 goroutine 并发处理任务,提升效率。使用 goroutine pool:创建一个 goroutine 池,为并发任务分配和管理 goroutine。cache 优化:通过缓存优化减少对数据库或其他慢速操作的调用,提高响应速度。
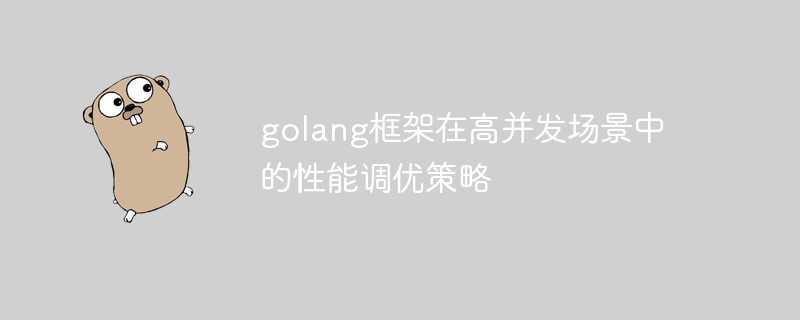
Golang 框架在高并发场景中的性能调优策略
简介
在高并发场景中,Golang 框架的性能至关重要。本文将探讨各种策略,以优化 Golang 框架在高并发环境下的性能。
立即学习“go语言免费学习笔记(深入)”;
代码段 1:并行处理
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
func main() {
pool := &sync.Pool{ New : func () interface {} { return new (Worker) }}
for i := 10 ; i >= 0 ; i-- {
w := pool.Get().(*Worker)
defer pool.Put(w)
go func (w *Worker, i int ) {
w.Do(i)
}(w, i)
}
}
type Worker struct {}
func (w *Worker) Do(i int ) {
}
|
代码段 2:使用 Goroutine Pool
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
package main
import (
"sync"
"runtime"
)
func main() {
var wg sync.WaitGroup
var pool = make ( chan func (), runtime.NumCPU()* 2 )
for i := 0 ; i < 1000 ; i++ {
wg.Add( 1 )
pool <- func () {
defer wg.Done()
}
}
close (pool)
wg.Wait()
}
|
代码段 3:Cache 优化
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
type Cache struct {
mu sync.Mutex
data map [ string ] interface {}
}
func (c *Cache) Get(key string ) ( interface {}, bool ) {
c.mu.Lock()
defer c.mu.Unlock()
if val, ok := c.data[key]; ok {
return val, true
}
return nil , false
}
func (c *Cache) Set(key string , val interface {}) {
c.mu.Lock()
defer c.mu.Unlock()
c.data[key] = val
}
|
实战案例
以上策略应用于一个大型电子商务网站。通过并行处理,Goroutine 池和缓存优化,网站的响应时间显著降低,吞吐量显著提高。
持续的性能调优至关重要,包括监控关键指标和对代码和架构进行持续的审查。