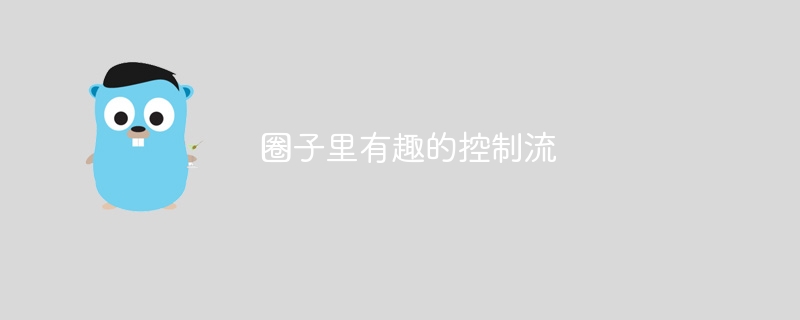
在 go (golang) 中,控制流是使用几个基本结构来管理的,包括条件语句(if、else)、循环(for)和 switch 语句。以下是这些构造在 go 中如何工作的概述:
- 条件:if、else、else if 在 go 中,if 语句用于根据条件执行代码。与其他一些语言不同,go 不需要在条件两边加上括号。然而,大括号 {} 是强制性的。
基本声明
1
2
3
4
5
6
7
8
9
10
11
|
package main
import "fmt"
func main() {
age := 20
if age >= 18 {
fmt.println( "you are an adult." )
}
}
|
‘if-else 语句’示例
`包主
导入“fmt”
func main() {
年龄 := 16
1
2
3
4
5
|
if age >= 18 {
fmt.println( "you are an adult." )
} else {
fmt.println( "you are not an adult." )
}
|
}
`
‘if-else if-else’ 语句:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
package main
import "fmt"
func main() {
age := 20
if age >= 21 {
fmt.println( "you can drink alcohol." )
} else if age >= 18 {
fmt.println( "you are an adult, but cannot drink alcohol." )
} else {
fmt.println( "you are not an adult." )
}
}
|
2.循环:for
go 使用“for”循环来满足所有循环需求;它没有“while”或循环
基本的“for”循环:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
package main
import "fmt"
func main() {
for i := 0; i
<p> 'for' 作为 'while' 循环:<br></p>
<pre class = "brush:php;toolbar:false" >package main
import "fmt"
func main() {
i := 0
for i
<p>无限循环:<br></p>
<pre class = "brush:php;toolbar:false" >package main
func main() {
for {
}
}
|
带有“range”的“for”循环:
这通常用于迭代切片、数组、映射或字符串。
1
2
3
4
5
6
7
8
9
10
11
|
package main
import "fmt"
func main() {
numbers := []int{1, 2, 3, 4, 5}
for index, value := range numbers {
fmt.println( "index:" , index, "value:" , value)
}
}
|
- switch 语句 go go 中的“switch”语句用于选择要执行的多个代码块之一。go 的“switch”比其他一些语言更强大,可以与任何类型的值一起使用,而不仅仅是整数。
基本“开关”
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
package main
import "fmt"
func main() {
day := "monday"
switch day {
case "monday" :
fmt.println( "start of the work week." )
case "friday" :
fmt.println( "end of the work week." )
default :
fmt.println( "midweek." )
}
}
|
在一个 case 中切换多个表达式:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
package main
import "fmt"
func main() {
day := "saturday"
switch day {
case "saturday" , "sunday" :
fmt.println( "weekend!" )
default :
fmt.println( "weekday." )
}
}
|
不带表达式的 switch 的作用就像一串 if-else 语句。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
package main
import "fmt"
func main() {
age := 18
switch {
case age = 18 && age
<ol>
<li>推迟、恐慌和恢复
</li>
</ol><pre class = "brush:php;toolbar:false" >package main
import "fmt"
func main() {
defer fmt.println( "this is deferred and will run at the end." )
fmt.println( "this will run first." )
}
|
恐慌与恢复
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
package main
import "fmt"
func main() {
defer func() {
if r := recover(); r != nil {
fmt.Println( "Recovered from panic:" , r)
}
}()
fmt.Println( "About to panic!" )
panic( "Something went wrong." )
}
|