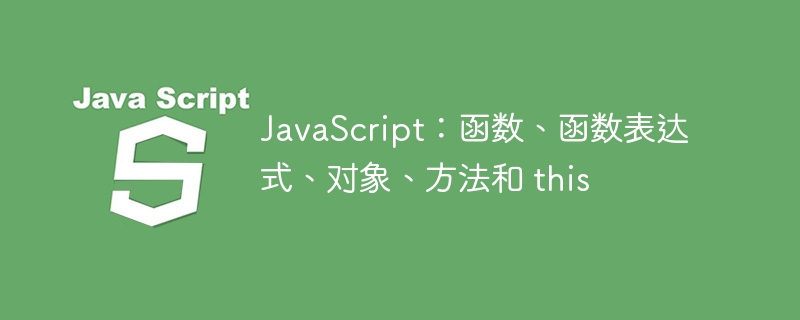
简单的基本功能
这是一个不带参数的简单函数:
1
2
3
4
5
|
function hello() {
console.log( 'hello there stranger, how are you?' );
}
hello();
|
这是一个带有一个参数的函数:
1
2
3
4
5
|
function greet(person) {
console.log(`hi there ${person}.`);
}
greet( 'megan' );
|
我们可以有多个参数,如下所示:
1
2
3
4
5
|
function greetfullname(fname, lname) {
console.log(`hi there ${fname} ${lname}.`);
}
greetfullname( 'megan' , 'paffrath' );
|
函数表达式
函数表达式只是编写函数的另一种方式。他们的工作方式仍然与上面相同:
1
2
3
4
5
|
const square = function (x) {
return x * x;
};
square(2);
|
高阶函数
这些函数与其他函数一起运行/在其他函数上运行,也许它们:
将另一个函数作为参数的函数的示例是:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
function calltwice(func) {
func();
func();
}
let laugh = function () {
console.log( 'haha' );
};
calltwice(laugh);
function rolldie() {
const roll = math. floor (math.random() * 6) + 1;
console.log(roll);
}
calltwice(rolldie);
|
函数返回函数的一个例子是:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
function makemysteryfunc() {
const rand = math.random();
if (rand > 0.5) {
return function () {
console.log( 'you win' );
};
} else {
return function () {
alert( 'you have been infected by a computer virus' );
while (true) {
alert( 'stop trying to close this window.' );
}
};
}
}
let returnedfunc = makemysteryfunc();
returnedfunc();
|
另一个(更有用的例子)是:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
function makebetweenfunc(min, max) {
return function (num) {
return num >= min && num
<h2>
方法
</h2>
<p>我们可以添加函数作为对象的属性(这些称为方法)。</p>
<p>例如:<br></p>
<pre class = "brush:php;toolbar:false" > const mymath = {
pi: 3.14,
square: function (num) {
return num * num;
},
cube(num) {
return num ** 3;
},
};
|
这
“this”主要在对象的方法中使用。它用于引用对象的属性。
1
2
3
4
5
6
7
8
9
10
11
|
const person = {
first: 'abby' ,
last: 'smith' ,
fullname() {
return `${this.first} ${this.last}`;
},
};
person.fullname();
person.lastname = 'elm' ;
person.fullname();
|
注意,在对象之外,“this”指的是顶级窗口对象。要查看其中包含的内容,请在控制台中输入。通用函数也存储在 this 对象中:
1
2
3
4
5
6
|
function howdy() {
console.log( 'HOWDY' );
}
this.howdy();
|