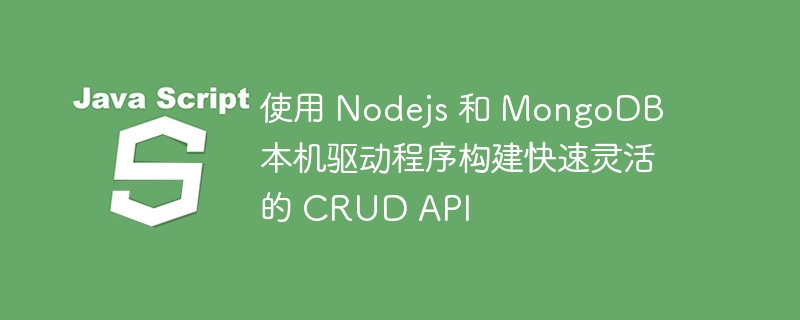
将 node.JS 和 Express 与 mongodb 本机驱动程序结合使用:原因和方式
如果您使用 node.js 和 express,您可能遇到过 mongoose,这是一个流行的 mongodb odm(对象数据建模)库。虽然 mongoose 提供了许多有用的功能,但您可能有理由选择直接使用 mongodb 的本机驱动程序。在这篇文章中,我将带您了解使用 mongodb 本机驱动程序的好处,并分享如何使用它们实现简单的 crud API。
为什么使用 mongodb 本机驱动程序?
-
性能:mongodb 原生驱动程序通过直接与 mongodb 交互来提供更好的性能,而无需 mongoose 引入的额外抽象层。这对于高性能应用程序特别有益。
-
灵活性:本机驱动程序可以更好地控制您的查询和数据交互。 mongoose 及其模式和模型强加了一些结构,这可能并不适合每个用例。
-
减少开销:通过使用本机驱动程序,您可以避免维护 mongoose 模式和模型的额外开销,这可以简化您的代码库。
点击下载“电脑DLL修复工具”;
-
学习机会:直接使用原生驱动可以帮助你更好地理解mongodb的操作,并且可以是一个很好的学习体验。
使用 mongodb 本机驱动程序实现 crud api
这是有关如何使用 node.js、express 和 mongodb 本机驱动程序设置简单 crud api 的分步指南。
1. 设置数据库连接
创建 utils/db.js 文件来管理 mongodb 连接:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
|
require ( 'do.env' ).config()
const dbconfig = require ( '../config/db.config' );
const { mongoclient } = require ( 'mongodb' );
const client = new mongoclient(dbconfig.url);
let _db;
let connectpromise;
async function connecttodb() {
if (!connectpromise) {
connectpromise = new promise(async (resolve, reject) => {
try {
await client.connect();
console.log( 'connected to the database ?' , client.s.options.dbname);
_db = client.db();
resolve(_db);
} catch (error) {
console.error( 'error connecting to the database:' , error);
reject(error);
}
});
}
return connectpromise;
}
function getdb() {
if (!_db) {
throw new error( 'database not connected' );
}
return _db;
}
function isdbconnected() {
return boolean(_db);
}
module.exports = { connecttodb, getdb, isdbconnected };
|
2. 定义你的模型
创建 models/model.js 文件来与 mongodb 集合交互:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
|
const { objectid } = require ( 'mongodb' );
const db = require ( '../utils/db' );
class appmodel {
constructor( $collection ) {
this.collection = null;
(async () => {
if (!db.isdbconnected()) {
console.log( 'waiting for the database to connect...' );
await db.connecttodb();
}
this.collection = db.getdb().collection( $collection );
console.log( 'collection name:' , $collection );
})();
}
async find() {
return await this.collection.find().toarray();
}
async findone(condition = {}) {
const result = await this.collection.findone(condition);
return result || 'no document found!' ;
}
async create(data) {
data.creatEDAt = new date ();
data.updatedat = new date ();
let result = await this.collection.insertone(data);
return `${result.insertedid} inserted successfully`;
}
async update(id, data) {
let condition = await this.collection.findone({ _id: new objectid(id) });
if (condition) {
const result = await this.collection.updateone({ _id: new objectid(id) }, { $set : { ...data, updatedat: new date () } });
return `${result.modifiedcount > 0} updated successfully!`;
} else {
return `no document found with ${id}`;
}
}
async deleteone(id) {
const condition = await this.collection.findone({ _id: new objectid(id) });
if (condition) {
const result = await this.collection.deleteone({ _id: new objectid(id) });
return `${result.deletedcount > 0} deleted successfully!`;
} else {
return `no document found with ${id}`;
}
}
}
module.exports = appmodel;
|
3. 设置路线
创建一个index.js文件来定义您的api路由:
1
2
3
4
5
6
7
8
9
10
11
12
|
const express = require ( 'express' );
const router = express.router();
const users = require ( '../controllers/usercontroller' );
router.get( "/users" , users.findall);
router.post( "/users" , users.create);
router.put( "/users" , users.update);
router.get( "/users/:id" , users.findone);
router. delete ( "/users/:id" , users.deleteone);
module.exports = router;
|
4. 实施控制器
创建一个 usercontroller.js 文件来处理您的 crud 操作:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
|
const { objectid } = require ( 'mongodb' );
const model = require ( '../models/model' );
const model = new model( 'users' );
let usercontroller = {
async findall(req, res) {
model.find()
.then(data => res.send(data))
. catch (err => res.status(500).send({ message: err.message }));
},
async findone(req, res) {
let condition = { _id: new objectid(req.params.id) };
model.findone(condition)
.then(data => res.send(data))
. catch (err => res.status(500).send({ message: err.message }));
},
create(req, res) {
let data = req.body;
model.create(data)
.then(data => res.send(data))
. catch (error => res.status(500).send({ message: error.message }));
},
update(req, res) {
let id = req.body._id;
let data = req.body;
model.update(id, data)
.then(data => res.send(data))
. catch (error => res.status(500).send({ message: error.message }));
},
deleteone(req, res) {
let id = new objectid(req.params.id);
model.deleteone(id)
.then(data => res.send(data))
. catch (error => res.status(500).send({ message: error.message }));
}
}
module.exports = usercontroller;
|
5. 配置
将 mongodb 连接字符串存储在 .env 文件中,并创建 db.config.js 文件来加载它:
1
2
3
|
module.exports = {
url: process.env.DB_CONFIG,
};
|
结论
从 mongoose 切换到 mongodb 本机驱动程序对于某些项目来说可能是一个战略选择,可以提供性能优势和更大的灵活性。此处提供的实现应该为您开始使用 node.js 和 mongodb 本机驱动程序构建应用程序奠定坚实的基础。
随时查看 Github 上的完整代码,并为您自己的项目探索更多功能或增强功能!
还有任何问题欢迎评论。