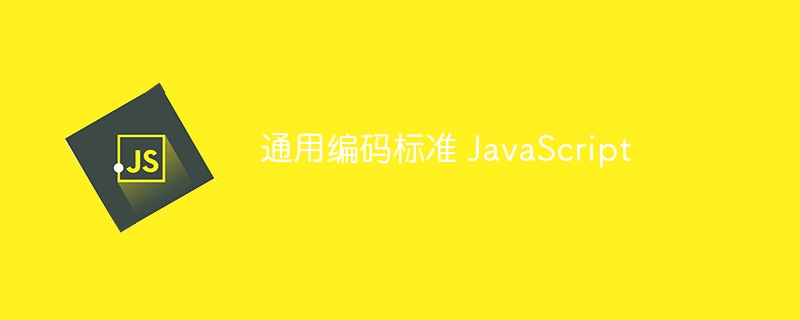
- 有意义的名字:
- 使用有意义且具有描述性的变量和函数名称。
- 除了循环计数器之外,避免使用缩写和单字母名称。
1
2
3
4
5
6
7
|
const userage = 25;
function calculatetotalprice(items) { ... }
const a = 25;
function calc(items) { ... }
|
- 一致的命名约定:
- 变量和函数使用驼峰命名法。
- 使用 pascalcase 命名类名。
1
2
3
|
const userage = 25;
function calculatetotalprice(items) { ... }
class userprofile { ... }
|
- 避免重复:
- 遵循 dry(don’t repeat yourself)原则,将重复的代码封装到函数中。
1
2
3
4
5
6
7
8
|
function calculatediscount(price, discount) { ... }
const price1 = calculatediscount(100, 10);
const price2 = calculatediscount(200, 20);
const price1 = 100 - 10;
const price2 = 200 - 20;
|
- 错误处理:
- 将 API 调用和其他异步操作包装在 try-catch 块中。
1
2
3
4
5
6
7
8
9
|
async function fetchdata() {
try {
const response = await fetch( 'api/url' );
const data = await response.JSon();
return data;
} catch (error) {
console.error( 'error fetching data:' , error);
}
}
|
- 边缘情况:
1
2
3
4
5
6
|
function getuserage(user) {
if (!user || !user.age) {
return 'age not available' ;
}
return user.age;
}
|
- 一致的格式:
- 遵循一致的代码格式风格(缩进、间距等)。使用 prettier 等工具来自动化此操作。
1
2
3
4
5
|
if (condition) {
dosomething();
} else {
dosomethingelse();
}
|
代码组织
- 模块化:
1
2
3
4
5
|
export function calculatediscount(price, discount) { ... }
import { calculatediscount } from './utils.js' ;
|
- 关注点分离:
- 将不同的关注点(例如,ui 逻辑、业务逻辑、数据处理)分离到不同的文件或函数中。
1
2
3
4
5
6
7
8
9
|
function updateui(data) { ... }
async function fetchdata() { ... }
import { updateui } from './ui.js' ;
import { fetchdata } from './data.js' ;
|
最佳实践
- 使用严格模式:
- 使用常量:
- 避免全局变量:
1
2
3
4
5
6
7
|
( function () {
const localvariable = 'this is local' ;
})();
var globalvariable = 'this is global' ;
|
- 评论和文档:
1
2
3
4
5
6
7
|
function calculatetotalprice(price, discount) { ... }
|
- 使用 promises 和异步/等待进行错误处理:
- 更喜欢使用 promise 和 async/await 进行异步操作,并将它们包装在 try-catch 块中以进行错误处理。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
async function fetchdata() {
try {
const response = await fetch( 'api/url' );
const data = await response.JSON();
return data;
} catch (error) {
console.error( 'error fetching data:' , error);
}
}
function fetchdata(callback) {
fetch( 'api/url' )
.then(response => response.json())
.then(data => callback(data))
. catch (error => console.error( 'error fetching data:' , error));
}
|
- 对象解构:
1
2
3
4
5
6
7
|
const vehicle = { make: 'Toyota' , model: 'Camry' };
const { make, model } = vehicle;
const vehicleMake = vehicle.make;
const vehicleModel = vehicle.model;
|
通过遵循这些标准,您可以确保您的 JavascrIPt 代码干净、可维护且高效。